An Introduction to Tweening With ActionScript 3.0

Flash is known for delivering rich interactive experiences with a lot of "eye-candy". Often, this eye-candy is achieved by means of animation and Flash has great animating capabilities. During this tutorial we'll discuss tweening; Flash's automated animation.
Introduction
In this tutorial I'll discuss the following:
- What is a tween?
- Using the Tween class.
- Tips & tricks for using the Tween class.
- Third party tweening classes.
Step 1: What Is a Tween?
Wikipedia explains tweening as the following:
Inbetweening or tweening is the process of generating intermediate frames between two images to give the appearance that the first image evolves smoothly into the second image. Inbetweens are the drawings between the keyframes which help to create the illusion of motion. Inbetweening is a key process in all types of animation, including computer animation.
Most people, who have ever used Flash, have encountered a tween before. Since the early beginning of Flash, there's been the possibility to tween by using the timeline.


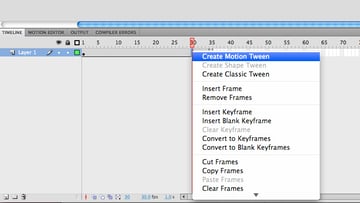
We won't be discussing how to tween using the timeline, we'll discuss how to tween using ActionScript 3.0!
Step 2: The Tween Class
The Tween class is the class which handles all the animation, and can animate all properties that have a numeric value. These properties could be:
- x position
- y position
- alpha (opacity)
- etc
Even though the Tween class is mostly used to animate display objects (objects that can be seen), you can use it just as effectively to tween (for example) the volume of a sound channel.
Step 3: Syntax of the Tween Class
The constructor of the Tween class is pretty lengthy, but don't worry, it's very simple.
1 |
var tween:Tween = new Tween(obj:Object, prop:String, func:Function, begin:Number, finish:Number, duration:Number, useSeconds:Boolean = false) |
Let's go through the parameters one by one. We want to fade in a movie clip named square as an example. OK, let's do this:
- The first parameter is the object that we'll be tweening, this could be a movie clip named square.
- The second parameter is the property that we'll be tweening. This property must be a string, so if we're tweening the alpha of our square we'd use "alpha".
- The third parameter is the transition that the tween will use. A transition gives us more control about how the tween will animate the property (eg.) that it gradually speeds up. We'll discuss transitions in the next step.
- The fourth parameter is the value that the property starts at. If we'd fade something in, then the alpha would start at 0 (or else we would already see the square).
- The fifth parameter is the value that the property ends with. We're fading our square in, so at the end the alpha will be 1 (1 alpha = 100% opacity).
- The sixth parameter is the duration of the tween. This can be in frames, or in seconds. By default the duration is measured in frames.
- The seventh and final parameter is a boolean. This boolean defines if we want to use seconds as the measurement of duration. If it's true, then the duration is measured in seconds. By default this parameter is false.
Step 4: Transitions
Transitions have already been mentioned in step 3, however let's go into more detail. A transition is a function, which describes how a property moves towards an end value. These transitions can also create certain effects, for example a bounce, or an elastic effect.
There are loads of transitions, and they've been split in to several classes as follows:
- Backthe transitions make the object go back, before tweening to the end value. Think of a catapult, it starts at 0, pulls back to -20 and afterwards shoots forward to 200.
- Bouncethe transitions make the object bounce.
- Elasticthe transitions make the object seem elastic.
- Nonethe transitions do nothing, the property reaches the end value in a constant motion.
- Regularthe transitions have a small build up to a constant speed. Think of a car, it must first accelerate before getting to speed.
- Strongthe transitions have a much larger build up to a constant speed. Think of a space shuttle, it also accelerates, but for much longer.
Step 5: easeIn, easeOut and easeInOut
So what do you do if you don't want to gradually accelerate, but gradually slow down? All transition classes have three functions:
- easeInthe transition affects the property at the beginning.
- easeOutthe transition affects the property at the end.
- easeInOutthe transition affects the property at the beginning and end.
These transitions can be drawn as a function (and with function I mean a mathematical function, a line). Look at this transition cheat sheet to get an idea of what each transition does. Some of the transitions haven't been mentioned, this is because these transitions are from the Tweener class, which I'll mention in step 18.
Just try the demo and check how each transition affects a property. This is the best way to get an idea of what transitions do to a tween.
Step 6: Our First Tween
So now let's finally get to work! We're going to create our very first tween. Draw a square and convert it to a movie clip, afterwards give it an instance name of square.



Now that we have our movie clip, let's create a tween! First we must import the necessary classes, because these aren't imported at runtime.
1 |
//import the Tween class
|
2 |
import fl.transitions.Tween; |
3 |
//import the transitions
|
4 |
import fl.transition.easing.*; |
Afterwards, create a variable named tween, of type Tween.
1 |
//import the Tween class
|
2 |
import fl.transitions.Tween; |
3 |
//import the transitions
|
4 |
import fl.transition.easing.*; |
5 |
|
6 |
//create a var tween
|
7 |
var tween:Tween; |
Now pass the parameters to the constructor. The values for our parameters are:
- The object we're tweening is square.
- The property we're tweening is x.
- The transition function we'll be using is: Regular.easeInOut.
- The starting position of square will be 0.
- The finishing position of square will be 400.
- The tween will last 1.5 seconds.
- So that means that useSeconds will be true.
Our code will look like this:
1 |
//import the Tween class
|
2 |
import fl.transitions.Tween; |
3 |
//import the transitions
|
4 |
import fl.transitions.easing.*; |
5 |
|
6 |
//create a var tween
|
7 |
var tween:Tween = new Tween(square, 'x', Regular.easeInOut, 0, 400, 1.5, true); |
If you test the flash movie (Control > Test Movie), you'll see the following:
Step 7: Understanding our App
Now it's time to build our demo. As this tutorial is about tweening, I won't discuss how to create the interface, just open step7.fla, which is located in the source files.
Before we even start writing one line of code, let's look how the file is built. There are 4 layers: Actions, UI, Rabbit and BG. We'll write all our code in the layer Actions. The UI layer has all our user interface components. Take a good look at all the instance names of all the components. The rabbit layer contains a movie clip with the instance name rabbit, we'll be tweening this movie clip. The BG layer simply contains a background, which will simply do nothing. We've gotta give our lovely bunny a nice place where he can do anything he wants to, right?
Step 8: Declaring Variables
A good practice is to first declare all the (global) variables that we will use. The code has been heavily commented, to give you an idea what each variable does.
Editor's note: I'm afraid the ActionScript in this step is causing our syntax highlighter to trip Firefox up (this sometimes happens and I've no idea why). For now, it's best you download it to have a look. Sorry for the inconvenience.
As you might have noticed, we're using a two-dimensional array. If you're not yet so experienced with Arrays, check Dru Kepple's amazing tutorial about arrays - AS3 101: Arrays. This two-dimensional array contains all possible transitions.
Step 9: Adding Event Listeners
Even though we have buttons, we need to add event listeners, or else our buttons won't do anything. If you don't understand how event listeners work, or want to learn about the event framework, check my other tutorial Taking a Closer Look at the ActionScript 3.0 Event Framework.
1 |
//when tweenButton is clicked, begin animating (fire the function animate)
|
2 |
tweenButton.addEventListener(MouseEvent.CLICK, animate); |
3 |
|
4 |
//when resetButton is clicked, reset all properties of rabbit (fire the function reset)
|
5 |
resetButton.addEventListener(MouseEvent.CLICK, reset); |
Step 10: Creating the Animate Function
When tweenButton is clicked, we want to start animating. To do this, we have to perform quite a few tasks:
- Retrieve all the values from all components.
- Set the correct values for transitionPos and easePos.
- Assign transition the correct function from the transitions array.
- Make tween call its constructor and tween rabbit.
1 |
//is called by the event listener attached to tweenButton
|
2 |
function animate(event:MouseEvent):void { |
3 |
//retrieve the time value from timeBox
|
4 |
time = timeBox.value; |
5 |
|
6 |
//retrieve the begin value from beginBox
|
7 |
beginValue = beginBox.value; |
8 |
|
9 |
//retrieve the end value from endBox
|
10 |
endValue = endBox.value; |
11 |
|
12 |
//retrieve the property from propertyBox
|
13 |
property = propertyBox.value; |
14 |
|
15 |
//set transition to the correct value
|
16 |
switch(transitionBox.value){ |
17 |
case "Back": |
18 |
transitionPos = 0; |
19 |
break; |
20 |
|
21 |
case "Bounce": |
22 |
transitionPos = 1; |
23 |
break; |
24 |
|
25 |
case "Elastic": |
26 |
transitionPos = 2; |
27 |
break; |
28 |
|
29 |
case "None": |
30 |
transitionPos = 3; |
31 |
break; |
32 |
|
33 |
case "Regular": |
34 |
transitionPos = 4; |
35 |
break; |
36 |
|
37 |
case "Strong": |
38 |
transitionPos = 5; |
39 |
break; |
40 |
}
|
41 |
|
42 |
//set ease to the correct value
|
43 |
switch(easeBox.value){ |
44 |
case "easeIn": |
45 |
easePos = 0; |
46 |
break; |
47 |
|
48 |
case "easeOut": |
49 |
easePos = 1; |
50 |
break; |
51 |
|
52 |
case "easeInOut": |
53 |
easePos = 2; |
54 |
break; |
55 |
}
|
56 |
|
57 |
//set transition to the precise transition in the transitions array
|
58 |
transition = transitions[easePos][transitionPos]; |
59 |
|
60 |
//tween!
|
61 |
tween = new Tween(rabbit, property, transition, beginValue, endValue, time, true); |
62 |
}
|
Step 11: Creating the reset function
When resetButton is clicked, we want to reset all the properties of rabbit. This is luckily much easier than creating the animate function.
1 |
function reset(event:MouseEvent):void { |
2 |
//reset all properties of rabbit
|
3 |
rabbit.alpha = START_ALPHA; |
4 |
rabbit.x = START_X; |
5 |
rabbit.y = START_Y; |
6 |
rabbit.rotation = START_ROTATION; |
7 |
rabbit.scaleX = START_SCALEX; |
8 |
rabbit.scaleY = START_SCALEY; |
9 |
rabbit.width = START_WIDTH; |
10 |
rabbit.height = START_HEIGHT; |
11 |
}
|
Step 12: Feel Proud of Yourself!
If you did everything correctly, then you'll have successfully built the demo. Congrats, you did it!
Download the whole code and admire all that we've written:
Step 13: Wait, There's More!
Of course, knowing how to tween isn't enough. We still need to discuss the following:
- How to tween multiple properties.
- How to create chains of tweens.
- How to have a delay before your tweens.
- Third party tweening classes.
All the following examples will be done with a movie clip, with an instance name of square.
Step 14: Starting Value
Sometimes it's great to set a starting value for a tween, however most times you'll want to tween from the object's current position. Just use the object's property as the starting value.
1 |
//import necessary classes
|
2 |
import fl.transitions.Tween; |
3 |
import fl.transitions.easing.*; |
4 |
|
5 |
var tween:Tween = new Tween(square, "alpha", square.alpha, .75, 1, true); |
Step 15: Multiple Properties
Tweening multiple properties is very easy to do, just use the constructor several times.
1 |
//import necessary classes
|
2 |
import fl.transitions.Tween; |
3 |
import fl.transitions.easing.*; |
4 |
|
5 |
var tween:Tween; |
6 |
tween = new Tween(square, "x", Regular.easeOut, 0, 400, 1.5, true); |
7 |
tween = new Tween(square, "y", Elastic.easeOut, 30, 200, 3.5, true); |
8 |
tween = new Tween(square, "rotation", Bounce.easeOut, 0, 90, 3, true); |
Step 16: Chain of Tweens
A chain of tweens is that there are multiple tweens, each one starting when the previous one finishes. We need to do this by using the event TweenEvent.MOTION_FINISH. This event occurs when a tween finishes tweening.
1 |
//import necesarry classes
|
2 |
import fl.transitions.Tween; |
3 |
import fl.transitions.easing.*; |
4 |
import fl.transitions.TweenEvent; |
5 |
|
6 |
//create the tweens
|
7 |
var tween1:Tween = new Tween(square, "x", Strong.easeInOut, 0, 400, 1.5, true); |
8 |
var tween2:Tween = new Tween(square, "y", Bounce.easeOut, square.y, 200, 1, true); |
9 |
var tween3:Tween = new Tween(square, "alpha", None.easeNone, square.alpha, 0, 2, true); |
10 |
|
11 |
//prevent tween2 and tween3 to start tweening
|
12 |
tween2.stop(); |
13 |
tween3.stop(); |
14 |
|
15 |
//add event listeners to tween1 and tween 2
|
16 |
tween1.addEventListener(TweenEvent.MOTION_FINISH, startTween2); |
17 |
tween2.addEventListener(TweenEvent.MOTION_FINISH, startTween3); |
18 |
|
19 |
//create the functions startTween2 and startTween3
|
20 |
function startTween2(event:TweenEvent):void { |
21 |
//make tween2 start
|
22 |
tween2.start(); |
23 |
}
|
24 |
|
25 |
function startTween3(event:TweenEvent):void { |
26 |
//make tween3 start
|
27 |
tween3.start(); |
28 |
}
|
You can also use the event TweenEvent.MOTION_FINISH for things other than creating a chain of tweens. Think of a flash site, after a page has been tweened, a function will be called, which will load text to display.
Step 17: Tweens with Delay
Creating a tween with a delay is very similar to creating a chain of tweens. We're using a Timer, and when the event TimerEvent.TIMER occurs, then the tween will start.
1 |
//import necesarry classes
|
2 |
import fl.transitions.Tween; |
3 |
import fl.transitions.easing.*; |
4 |
|
5 |
//create our timer
|
6 |
var timer:Timer = new Timer(3000); |
7 |
|
8 |
//start the timer
|
9 |
timer.start(); |
10 |
|
11 |
//create our tween
|
12 |
var tween:Tween = new Tween(square, "x", Strong.easeInOut, square.x, 300, 2, true); |
13 |
|
14 |
//prevent tween from immediately tweening
|
15 |
tween.stop(); |
16 |
|
17 |
//add an event listener to timer
|
18 |
timer.addEventListener(TimerEvent.TIMER, startTween); |
19 |
|
20 |
//create the function startTween
|
21 |
function startTween(event:TimerEvent):void { |
22 |
//make tween start
|
23 |
tween.start(); |
24 |
}
|
Step 18: Check the Documentation
The Tween class has quite a few methods, for example the yoyo function makes the tween play in reverse. So do look at the documentation about the Tween class.
Step 19: Third Party Tweening Classes
Sometimes the Tween class is enough for your project, however using the Tween class can be quite a hassle if you're going to tween a lot of properties, have a lot of delays etc.
Luckily there are several (free) third party classes, which have much more advanced tweening capabilities. Let's look at the pros and cons of using a third party class.
The pros:
- Development time is much faster.
- Tweening occurs much more efficiently than the Tween class. Performance will usually be improved while using third party classes.
The cons:
- As these classes aren't built in, you need to import them. This takes space and your flash project will need to load more (do note that currently these classes are extremely small.
- Some classes don't have a good documentation.
The most commonly used third party tweening classes are:
I won't discuss how to use each class, just check the links and find out which works best for you.
Thank you
Thank you for reading this tutorial., I hope it's given you a thorough understanding of how to use the Tween class. It was a pleasure to write for Flashtuts+! Why not follow me on Twitter, @DanielApt?